Each request sent to Hilton’s APIs must include information that identifies the Client AppYour application, which will be sending requests to the Hilton API.
(Synonym(s) - API consumer, client application, mobile app, web app, application name). This enables the API to verify (i.e., authenticate) the identity of the calling application. Hilton offers authentication by sending the request with the Bearer token. If you have any questions about authentication, please contact your API Coach.
This identification is controlled by an Access Token that is embedded into each API request header. The API will take the access token from the request, make sure that it is valid, and map it to the associated account.
Hilton's Authentication model is based on OAuth 2.01.
Authentication Flow Overview
Before you can connect to Hilton's APIs, you must have your application's Client IDA unique identifier for your Client App that is making requests to the Hilton API. This ID will be provided to you by Hilton.
(Synonym(s) - Consumer Key) and Client SecretA secret passphrase that is used together with the assigned Client ID to prove that your Client App is authorized to make a request.
(Synonym(s) - Consumer Secret, Secret) (i.e, your credentials).
Once you have your credentials, there are just 2 steps:
- Generate a Temporary Access Token using the Credentials.
- Submit Client ID and Client Secret to the Realms API for validation, and receive a temporary Access Token.
- Generate a Platform API Request using the Access Token
- Submit a platform API request which must include the temporary Access Token received in the previous step.
And we've also provided a few "Tips For Handling Authentication Failures" and a flow of the refresh process.
Have Your Client App's Credentials
You need two data elements to generate a valid access token – a client ID and a client secret. The client ID and client secret provide the ability to create temporary API access tokens on behalf of your application.
For functionality you have access to already, use your assigned client ID and client secret. Note, you need to use your Staging credentials to connect to STG (kapip-s) and your Production credentials to connect to PRD (kapip). Credentials are environment specific.
If you are existing Hilton partner who is adding functionality and need access to an area not available to your Client App, please work with your existing Hilton sponsor to request the additional access needed.
Generate a Temporary Access Token using the Credentials
The Realms API provides a temporary access token to allow your Client App to access Hilton's API. Send an authentication request to the Realms API (with valid credentials, of course), and an Access Token will be provided in response. The endpoint for the Realms API is /realms/applications/token
For example, to generate an access token for the staging environment, the URL would be:
https://kapip-s.hilton.io/hospitality-partner/v2/realms/applications/token
Here's a more robust example of the request and the response:
POST /hospitality-partner/v2/realms/applications/token
Host: kapip-s.hilton.io
Content-Type: application/json
Accept: application/json
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET"
}
This request, if successful, will return the access token:
{
"access_token": "8f59d3f20d3a8861ec2a083b7a09643d",
"scope": "am_application_scope default",
"token_type": "Bearer",
"expires_in": 3600
}
The access token can then be used to make an API call on behalf of your application.
The endpoints you are allowed to call will depend on the needs of your application and your agreement with Hilton.
- Use the same request (
/realms/applications/token
) again to request a new access token when the current one expires. - The expires_in value is provided in seconds (e.g., the 3600 in the example above means the access token will expire in 1 hour (i.e, 3600 seconds = 60 minutes = 1 hour).
- When your access token expires, the response code of 401 Unauthorized with a response payload similar to the following will be received:
{
"fault": {
"code": 900901,
"message": "Invalid Credentials",
"description": "Access failure for API: /hospitality-partner/v2, version: v2. Make sure you have given the correct access token"
}
}
Generate an API Request Using the Access Token
Send a request to the Platform API, with the access token obtained earlier (e.g. 8f59d3f20d3a8861ec2a083b7a09643d
) in the Authorization request header, to make an application-level API request. For example:
GET /hospitality-partner/v2/dcshop/props
Content-Type: application/json
Accept: application/json
Authorization: Bearer 8f59d3f20d3a8861ec2a083b7a09643d
The Authorization header must be in the form of
Authorization: Bearer YOUR ACCESS TOKEN GOES HERE
Tips For Handling Authentication Failures
If the request is not valid, the response returns the following error message:
{
"error_description":"Missing parameters: client_secret",
"error":"invalid_request"
}
If the request is not authorized due to invalid client id / client secret values, the response includes the following error message:
{
"error_description":"Client Authentication failed",
"error":"invalid_client"
}
- response code "401 - not authorized" signifies that there is a bad client id / client secret
- response code "403 - forbidden" signifies that the credentials do not allow access to that specific endpoint
- response code "503 - service unavailable" signifies that the Realms service is down
Writing Refresh Support Into Your Client App
The diagram below shows the overall workflow that your client app should use to- obtain an access token
- detect if it has expired
- refresh the access token when needed:
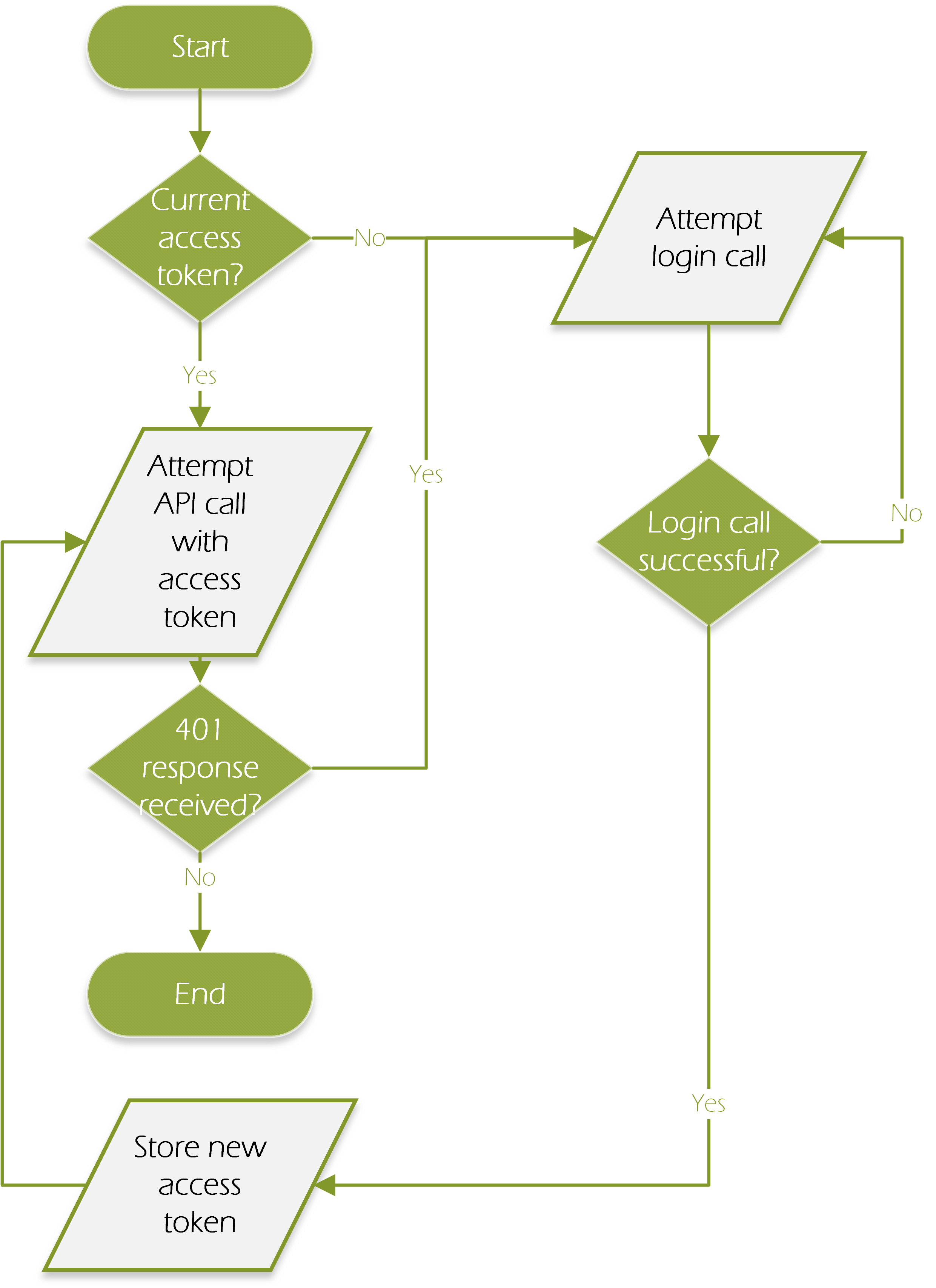